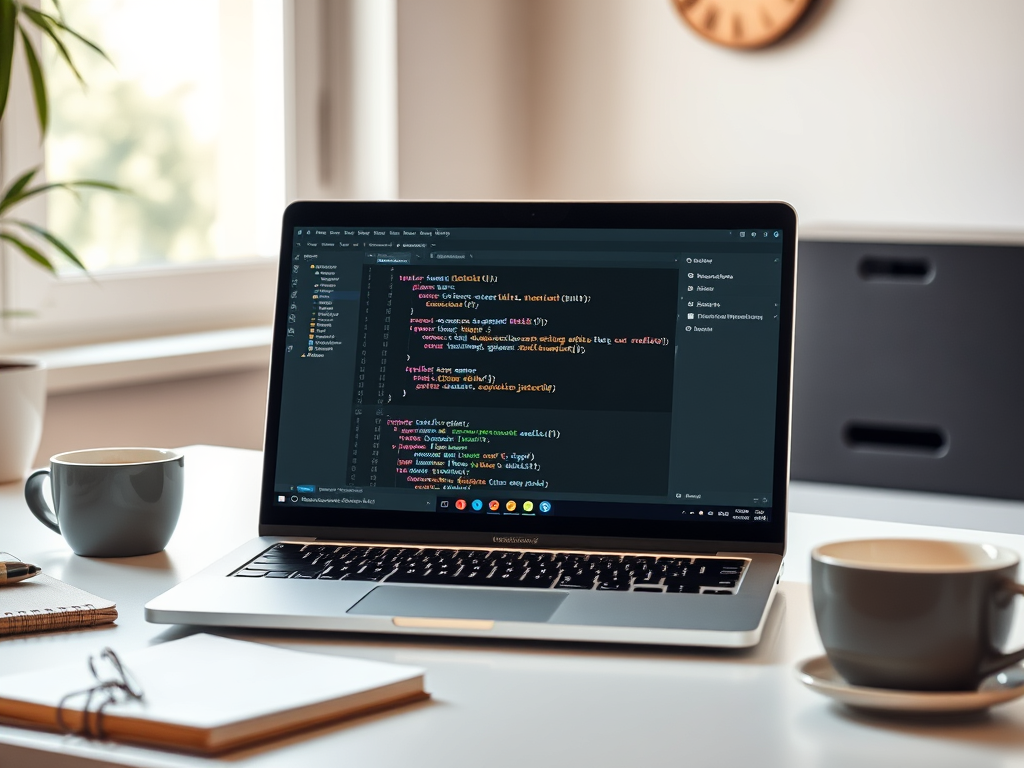
How to implement Android Push Notification

By, projscope.com
- 19 Mar, 2025
- 46 Views
- 0 Comment
In this tutorial, we’ll walk through an Android push notification app written in Kotlin. This guide will provide a step-by-step explanation on how to create a simple app and start using Dev-Sandbox infrastructure for real-time communication.
We’ve already built and tested a working app, which you can find and download from our repository. For this tutorial, we’ll be using Android Studio and an Android Emulator running API 35.
Quick start
- Download the app from our repository.
- Open it in Android Studio and allow it to install all dependencies.
- Once setup is complete, we’ll go over the key code changes to help you implement similar functionality in your own apps.
Understanding dependencies
implementation("com.microsoft.signalr:signalr:8.0.0")
This library, found in the build.gradle.kts file, is a wrapper over WebSockets, making real-time communication simpler and more efficient for developers.plugins {
alias(libs.plugins.android.application)
alias(libs.plugins.kotlin.android)
}
android {
namespace = "com.projscope.real_time_communication"
compileSdk = 35
defaultConfig {
applicationId = "com.projscope.real_time_communication"
minSdk = 35
targetSdk = 35
versionCode = 1
versionName = "1.0"
testInstrumentationRunner = "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
isMinifyEnabled = false
proguardFiles(
getDefaultProguardFile("proguard-android-optimize.txt"),
"proguard-rules.pro"
)
}
}
compileOptions {
sourceCompatibility = JavaVersion.VERSION_1_8
targetCompatibility = JavaVersion.VERSION_1_8
}
kotlinOptions {
jvmTarget = "1.8"
}
}
dependencies {
implementation(libs.androidx.core.ktx)
implementation(libs.androidx.appcompat)
implementation(libs.material)
implementation(libs.androidx.activity)
implementation(libs.androidx.constraintlayout)
implementation("com.microsoft.signalr:signalr:8.0.0") <---- SingalR library
testImplementation(libs.junit)
androidTestImplementation(libs.androidx.junit)
androidTestImplementation(libs.androidx.espresso.core)
}
Why SignalR?
- Provides an easy-to-use WebSocket-based communication layer.
- Handles automatic reconnection and message delivery failures.
- Great for real-time updates, making it perfect for notifications.
Establishing a SignalR Connection
The provided MainActivity.kt code looks great for starting and stopping the SignalR connection. Here’s a breakdown of how it works:
MainActivity Explanation
- SignalRService: This class handles the connection logic for SignalR, which is responsible for establishing and stopping the connection with the backend server.
- Start Button: When the user clicks the Start button (btn_start), it initiates the connection to the server using the startConnection() method from the SignalRService.
- Stop Button: When the user clicks the Stop button (btn_stop), it calls stopConnection() from SignalRService to disconnect from the server.
MainActivity Code Walkthrough:
package com.projscope.real_time_communication
import android.os.Bundle
import android.widget.Button
import androidx.activity.enableEdgeToEdge
import androidx.appcompat.app.AppCompatActivity
import androidx.core.view.ViewCompat
import androidx.core.view.WindowInsetsCompat
class MainActivity : AppCompatActivity() {
// Initialize the SignalRService to handle connection logic
private val signalRService = SignalRService()
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
enableEdgeToEdge() // Enables edge-to-edge UI for a full-screen experience
setContentView(R.layout.activity_main) // Set the activity layout
// Find the Start and Stop buttons by their IDs
val startButton: Button = findViewById(R.id.btn_start)
val stopButton: Button = findViewById(R.id.btn_stop)
// When the Start button is clicked, initiate connection
startButton.setOnClickListener {
signalRService.startConnection(baseContext) // Initiates connection with SignalR
}
// When the Stop button is clicked, stop the connection
stopButton.setOnClickListener {
signalRService.stopConnection() // Stops the connection with SignalR
}
// Handle system insets for edge-to-edge UI
ViewCompat.setOnApplyWindowInsetsListener(findViewById(R.id.main)) { v, insets ->
val systemBars = insets.getInsets(WindowInsetsCompat.Type.systemBars())
v.setPadding(systemBars.left, systemBars.top, systemBars.right, systemBars.bottom) // Adjust padding for system bars
insets // Return the insets
}
}
}
The SignalRService.kt you’ve shared is handling the real-time communication with the SignalR Hub and notifications for receiving messages. Let’s break down what this service does and walk through the core components.
SignalRService Explanation
- Establishing SignalR Connection: The startConnection method initializes the SignalR connection and connects to the hub at wss://api.dev-sandbox.dev/com-hub.
- Handling Notifications:
- When a message is received from the server (via ReceiveMessage event), it triggers a notification on the device.
- A notification channel is created, and heads-up notifications are shown for incoming messages.
- Sending Messages: The sendMessage method allows you to send messages to the server, which is useful for bi-directional communication.
Code Walkthrough - start connection
@SuppressLint("CheckResult")
fun startConnection(baseContext: Context) {
hubConnection = HubConnectionBuilder.create("wss://api.dev-sandbox.dev/com-hub")
.build()
context = baseContext
val notificationHelper = NotificationHelper(context)
notificationHelper.createNotificationChannel()
// Start the connection
hubConnection?.start()?.subscribe({
Log.d("SignalR", "Connected successfully!")
// Join Group
hubConnection?.invoke("JoinGroup", "api_;4+ospGZs]", "channel_AIuO1NqAeNuf")
Log.d("SignalR", "Joining group")
}, { error ->
Log.e("SignalR", "Error connecting: ${error.message}")
})
}
- SignalR Hub Connection: The connection is established to the SignalR Hub using HubConnectionBuilder.create(“wss://api.dev-sandbox.dev/com-hub”). It’s a WebSocket-based connection (wss), ensuring real-time communication.
- Handling Notifications:
- NotificationHelper is used to handle notification creation and management (including channel creation). This ensures that when a message is received, the app can send a heads-up notification.
- Connection Start: The connection is initiated with .start(), and once it successfully connects, the client joins a group with hubConnection?.invoke(“JoinGroup”, …) for communication.
- In case of an error while connecting, it logs an error message
Important!
Please note that the API key from your profile remains the same for all channels. You can obtain the channel identifier from the Channels Dashboard.
Code Walkthrough - Listening for Incoming Messages
// Listen for messages from the server
hubConnection?.on("ReceiveMessage", { message: String ->
Log.d("SignalR", "Received: $message")
val notificationTitle = "Realtime message"
val notificationContent = message
notificationHelper.showHeadsUpNotification(notificationTitle, notificationContent)
}, String::class.java)
- Event Listener: The code listens for incoming messages through the “ReceiveMessage” event.
- When a message is received, it logs the message and then triggers a notification using notificationHelper.showHeadsUpNotification().
Sending Messages
fun sendMessage(user: String, message: String) {
hubConnection?.invoke("SendMessage", user, message)
}
This method allows sending messages from the client to the server using the SendMessage event, which will trigger server-side logic on the backend.
NotificationHelper class
The NotificationHelper class is responsible for displaying popup notifications when a websocket notification is received. It creates a notification channel for Android 8.0+ and shows heads-up notifications with dynamic title and content. This ensures real-time updates are immediately visible to users, even when the app is in the background.
In summary
Our walkthrough will help you easily implement real-time communication using our API. By following the step-by-step guide, you’ll be able to set up your Android app with SignalR for websocket communication, configure push notifications, and integrate our API smoothly. The clear examples and practical code snippets will allow you to quickly establish a reliable connection and handle real-time messaging, ensuring a seamless experience for both you and your users.
This article can be found in one of our projects documentation – here.
Recent Comments
Category
- Android (1)
- Blog (5)
- Cloud Solutions (1)
- Software (3)
- Web development (2)